To create a production build you need to run the command npm run build
. It will create the dist
folder, that contains the production build files. Now you just need to upload the dist
files on to the server.
Static Server:
For environments using Node, the easiest way to handle this would be to install serve and let it handle the rest:
npm install -g serve
serve -s build
Other Solutions:
You don’t necessarily need a static server in order to run a project in production. It works just as fine integrated into an existing dynamic one.
Here’s a programmatic example using Node and Express:
const express = require('express');
const path = require('path');
const app = express();
app.use(express.static(path.join(__dirname, 'build')));
app.get('/', function (req, res) {
res.sendFile(path.join(__dirname, 'build', 'index.html'));
});
app.listen(9000);
Apache HTTP server:
If you’re using Apache HTTP Server, you need to create a .htaccess
file in the public
folder that looks like this:
Options -MultiViews
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-f
RewriteRule ^ index.html [QSA,L]
Upload the dist
files in the public folder.
Building for Relative Paths:
If you want to make your production build in different folder or sub directory. Like, if you want that the template run on the test.com/dist
rather then test.com
then you need to follow the steps given below:
Step 1: Open .webpack.config.js
file and search for
const publicPath = '/';
and set your path as per requirement like:
const publicPath = '/dist/';
Step 2: Now go to src>App.js
file and set the Router basename
to dist
like following code:
<Router basename={'/dist'}>
<Switch>
<Route path="/" component={App} />
</Switch>
</Router>
Step 3: Open .htaccess
file and set the roots for subdirectory: We have added the screenshot for the .htaccess
file location and also the code of the file.
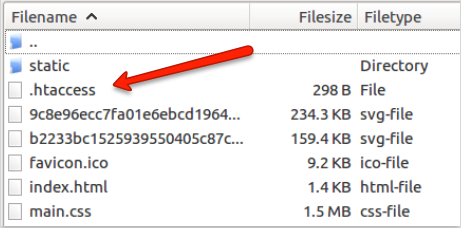
# To be inside the /Directory
<IfModule mod_rewrite.c>
RewriteEngine On
RewriteBase /dist/
RewriteRule ^index\.html$ - [L]
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule . /dist/index.html [L]
</IfModule>