We will help you in creating a new component. Follow the steps below to add a new component(example: test component):
Step 1: Create a new folder in src->views
. Ex: As we have created a test folder inside src->views
folder.
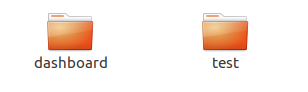
Step 2: In test folder, create a file named with the FolderName.vue. Ex: test.vue.
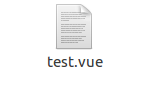
Step 3: Place the given code inside the file you have created in the above step. Ex: In test.vue file.
<template>
<div>
<page-title-bar></page-title-bar>
<h1>Test is Working</h1>
</div>
</template>
Step 4: We have to link the newly created component in the menu. Go to src->store->modules->sidebar->data.js
file. When you open the file, you will see the code structure like this:
// Sidebar Routers
export const menus = {
'message.general': [
{
action: 'zmdi-view-dashboard',
title: 'message.dashboard',
active: true,
items: [
{ title: 'message.ecommerce', path: '/default/dashboard/ecommerce', exact: true },
{ title: 'message.webAnalytics', path: '/mini/dashboard/web-analytics', exact: true },
{ title: 'message.magazine', path: '/horizontal/dashboard/magazine', exact: true },
{ title: 'message.news', path: '/boxed-v2/dashboard/news', exact: true },
{ title: 'message.agency', path: '/boxed/dashboard/agency', exact: true },
{ title: 'message.saas', path: '/horizontal/dashboard/saas', exact: true }
]
}
]
…
}
Add the link for newly created component in the menus object. Now your file will looks like this:
// Sidebar Routers
export const menus = {
'message.general': [
{
action: 'zmdi-view-dashboard',
title: 'message.dashboard',
active: true,
items: [
{ title: 'message.ecommerce', path: '/default/dashboard/ecommerce', exact: true },
{ title: 'message.webAnalytics', path: '/mini/dashboard/web-analytics', exact: true },
{ title: 'message.magazine', path: '/horizontal/dashboard/magazine', exact: true },
{ title: 'message.news', path: '/boxed-v2/dashboard/news', exact: true },
{ title: 'message.agency', path: '/boxed/dashboard/agency', exact: true },
{ title: 'message.saas', path: '/horizontal/dashboard/saas', exact: true }
]
}
],
// This is the new object you have to create for your new component.
'message.testpages': [
{
action: 'zmdi-view-dashboard',
title: 'message.test',
active: false,
items: [
{ title: 'message.test', path: '/test'},
]
}
]
}
The description of the keys used in the code are:
- action is icon
- title is page title
- active is state(true in dashboard case only, false in this case)
- items is an array of sub menu(if there is no sub menu then set it to null)
There are few things you need to take care about:
- State is always set to false in new components, It is true in dashboard case only.
- If there exists some sub menu items then pass it in the form of array to items. Make Sure the items of new component only contains title & path, not the exact key.
- If there is no sub menu, then the value of items key is set to null
items: null
. - If items is null then you have to pass the path in the main object like below:
{ action: 'zmdi-view-dashboard', title: 'message.test', active: false, items: null, path: '/test', }
Step 5: Add the route for the newly created component. Follow the given instructions to add the route. Open the src->router->default.js
file. Import the test component like below to use it in the rest of the file:
const test = () => import('Views/test/test');
Now add the component in the children array:
export default {
path: '/',
component: Full,
redirect: '/default/dashboard/ecommerce',
children: [
...
{
component: test,
path: '/default/test',
meta: {
requiresAuth: true,
title: 'message.test',
breadcrumb: 'Test'
}
}
]
}
If you choose a different layout for your template, then you have to follow the above mentioned step for that particular layout also. Make sure to add the path accordingly.
Now save the file and open the browser, you will see your added component in the template like below:
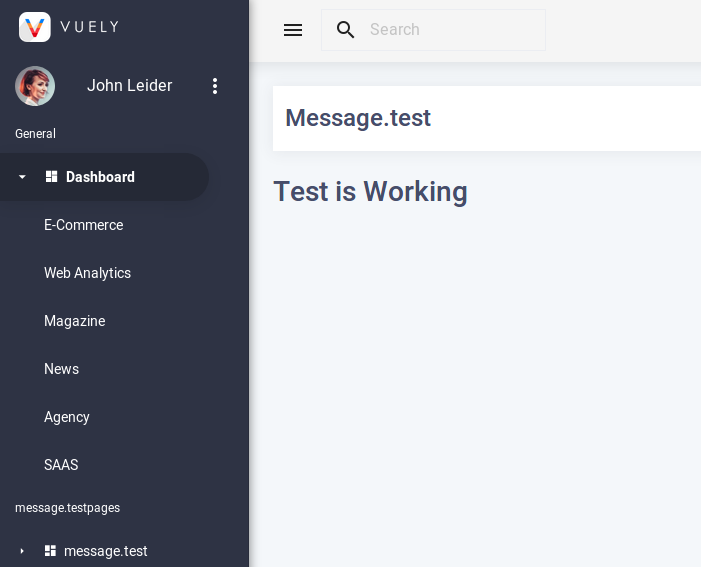
Don’t panic about the strings like message.testpages or message.test. We need to create these strings in the index.js
file in the src->lang->en
folder like below:
export default {
-------
-------
test: "Test",
testpages:"My Page"
}
Save this file and open the browser, your strings would be replaced by the message you have provided in the index.js
file.
Make sure if you are using any other language in the template then you will define the strings in that language folder also.
Now, You have successfully created a new component(named: test) in the template.